How to add CSS to HTML (With Link, Embed, Import, and Inline styles)
19 Feb 2009 — Updated 12 Mar 2023
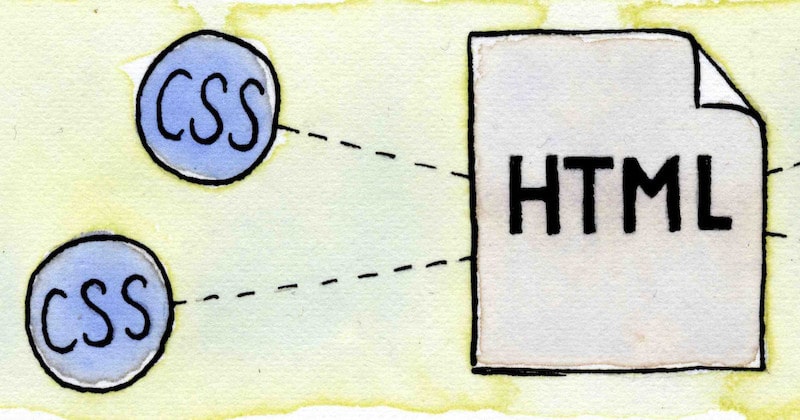
Table of contents
Adding CSS to HTML can be confusing because there are many ways to do it.
CSS can be added to HTML by linking to a separate stylesheet file, importing files from existing stylesheets, embedding CSS in a style tag, or adding inline styles directly to HTML elements. Many of these methods can also be done with javascript.
Today we're going to explore the pros and cons of each CSS method, see how they work, and learn when to use each one.
We'll also cover some of the common questions developers have when inserting CSS into HTML documents:
Let's get started.
1. Link to a Stylesheet File
This is the most common method of attaching CSS rules to HTML documents.
With this method, all your style rules are contained in a single text file that's saved with the .css extension. This file is saved on your server and you link to it directly from each HTML file. We use a link
tag which is a simple line of HTML that you put in the head
section of your HTML document, it looks like this:
<link rel="stylesheet" type="text/css" href="mystyles.css" media="screen" />
The rel
attribute is set to stylesheet
to tell the browser that the linked file is a Cascading Style Sheet (CSS).
If you're using HTML5, the type
attribute is not required, you can remove it and save a few bytes per page.
The href
attribute is where you specify the path to your CSS file.
If the CSS file is in the same folder as your HTML file then no path is required, you only need to specify the filename like in the example above.
If it's saved in a folder, then you can specify the folder path relative to the HTML file like this:
<link href="foldername/mystyles.css" ... >
You can also specify a path relative to the root of your domain by prefixing with a forward slash like this:
<link href="/foldername/mystyles.css" ... >
Absolute URLs also work:
<link href="https://www.yourdomain.com/foldername/mystyles.css" ... >
The media
attribute in a link tag specifies when the CSS rules are to be applied. Here are the most common values:
screen
indicates for use on a computer screen.projection
for projected presentations.handheld
for handheld devices (typically with small screens).print
to style printed web pages.all
(default value) This is what most people choose. You can leave off the media attribute completely if you want your styles to be applied for all media types.
Advantages of linking to a separate CSS file
Changes to your CSS are reflected across all pages: You only need to make a CSS change once in your single CSS file and all website pages will update.
Changing your website theme is easy: You can replace your CSS file to completely change the look of your website. Check out CSS Zen Garden for the best example of this.
Site speed increases for multiple page requests: When a person first visits your website their browser downloads the HTML of the current page plus the linked CSS file. When they navigate to another page, their browser only needs to download the HTML of the new page, the CSS file is cached so doesn't need to be downloaded again. This can make a big difference particularly if you have a large CSS file.
Disadvantages
- An additional HTTP request is required for each linked CSS file: Excess HTTP requests can delay the rendering of your page. We'll cover the solution to this problem shortly.
You can include as many CSS files in your HTML document as you like by adding multiple link tags, just remember, each file requires an additional HTTP request.
2. Embed CSS With a Style Tag
You can embed CSS rules directly into HTML documents by using a style
tag. Here's what this looks like:
<style type="text/css" media="screen">
/* Add style rules here */
</style>
Similar to the link tag, the type
attribute can be omitted for HTML5, and the media
value controls when your styles are applied (leave it off to default to all devices).
Add your CSS rules between the opening and closing style tags and write your CSS the same way as you do in stand-alone stylesheet files.
CSS rules are render-blocking so it's recommended to add style tags into the <head>
of the HTML document so they download as soon as possible. We'll discuss render-blocking CSS shortly.
Advantages of embedded style tags
Faster loading times: Because the CSS is part of the HTML document, the whole page exists as just one file. No extra HTTP requests are required. I use this method on my responsive columns demo layouts so when people view the source of the page they can see the HTML and the CSS code together.
Works great with dynamic styles: If you're using a database to generate page content you can also generate dynamic styles at the same time. Blogger does this by dynamically inserting the colors for headings and other elements into the CSS rules embedded in the page. This allows users to change these colors from an admin page without actually editing the CSS in their blog templates.
Disadvantages
- Embedded styles must be downloaded with every page request: These styles cannot be cached by the browser and re-used for another page. Because of this, it's recommended to embed a minimal amount of CSS as possible.
3. How to Add Inline Styles to HTML Elements With the Style Attribute
Style rules can be added directly to any HTML element. To do this, simply add a style attribute to the element then enter your rules as a single line of text (a string of characters) for the value.
Here's an example of a heading with inline styles:
<h2 style="color:red; background:black;">
This is a red heading with a black background
</h2>
Advantages of inline styles
Inline styles override external styles: This is because inline styles have a higher specificity than external CSS.
Faster pages: Just like embedded CSS, no extra HTTP requests are required.
You can change styles without editing the main CSS file: Sometimes you might need to change a style rule but you don't have access to the main website stylesheet, with this method you can add rules directly to each element instead.
Great for dynamic styles: For example, you can add a background-image URL as an inline style if it's different for each element.
Useful for HTML emails: EDMs (Electronic Direct Marketing) can be tricky to get right in all email clients, often the best way is to use inline styles everywhere.
Disadvantages
Excess styles can bloat your page: If you're specifying the same styles over and over your page weight will grow.
Maintenance can be tricky: Site-wide style changes will need to be made on every page, this can be tedious.
4. Load a Stylesheet File With the @import
Rule
Another interesting way to add CSS to an HTML page is with the @import
rule. This rule lets us attach a new CSS file from within CSS itself. Here's how this looks:
@import "newstyles.css";
Just change "newstyles" to the name of your CSS file and be sure to include the correct path to the file too. Remember the path is relative to the current CSS file that we are in, if the CSS is embedded into the HTML page then the path is relative to the HTML file.
Advantages of the @import
rule
- Adding new CSS files without changing HTML markup: Let's imagine we have a 1000 page website and we link to a CSS file from every page on the site. Now let's imagine we want to add a second CSS file to all of those pages. We could edit all 1000 HTML files and add a second CSS link or we could import the second CSS file from within the first file. We just saved ourselves many hours of work!
Disadvantages
Extra HTTP requests required: Every imported CSS file requires an additional HTTP request which can slow down page rendering.
Slow serial HTTP requests: If you import a CSS file from an existing external CSS file then the browser cannot begin downloading the second file until after it has received the first file and read its contents. You want to avoid serial CSS requests whenever possible.
5. Inject CSS With Javascript
Sometimes we need to apply CSS from within Javascript. We can do this in the following ways:
Inject an external stylesheet file with javascript
To do this we create a link element, add our CSS file path as the href attribute value, then inject it into the page with javascript:
/* create the link element */
const linkElement = document.createElement('link');
/* add attributes */
linkElement.setAttribute('rel', 'stylesheet');
linkElement.setAttribute('href', 'mystyles.css');
/* attach to the document head */
document.getElementsByTagName('head')[0].appendChild(linkElement);
Want to inject CSS into a shadow DOM? See the instructions in my article: Style Blocker: How To Prevent CSS Cascade With Shadow DOM
Insert a block of rules into a page with javascript
This method creates a style element, inserts our CSS rules as a string, then attaches the element to the HTML head.
/* specify our style rules in a string */
const cssRules = 'h1 { color:black; background:red; }';
/* create the style element */
const styleElement = document.createElement('style');
/* add style rules to the style element */
styleElement.appendChild(document.createTextNode(cssRules));
/* attach the style element to the document head */
document.getElementsByTagName('head')[0].appendChild(styleElement);
Add inline styles to individual elements with javascript
In this method we first get a handle on the element(s) we want to change, then we add CSS properties.
/* get a handle on the element */
const element = document.querySelector('h2');
/* add css properties */
element.css.color = 'blue';
element.css.backgroundColor = 'yellow';
Important note: In Javascript, multi-word CSS properties have their hyphens replaced with camel case, so background-color
becomes backgroundColor
(notice the capital 'C').
CSS and Page Performance
So now we've covered all the methods of adding CSS to HTML the next step is to learn how to put them all together and improve your website speed.
How to minify CSS to speed up your website
CSS in its hand-written state is quite verbose. We can reduce its file size by a process of minification.
What is CSS minifying?
Minifying CSS is the process of removing redundant data without affecting the browser's rendered output, this includes removing whitespace, line breaks, comments, unused code, and switching to shorthand properties to save space.
I recommend using CSS minifyer, it's a free online tool. Just make sure you keep a copy of your unminified CSS as your source code.
How to Inline Your Above-The-Fold CSS to Improve Page Rendering Time
If you want your page to load fast then you need to prioritize your above-the-fold content.
Above-the-fold content is any content that is visible in the viewport before you scroll down the page, naturally, this will be different for different sized devices and screens.
There is no exact answer to where the 'fold' is — you need to decide how far down the page is right for you based on the screen sizes of your website visitors. Check your site statistics for insights on this.
Once you've made a call on your page fold, identify all elements that appear above it, then inline that CSS in a style tag.
Next, link to your main stylesheet in a non-render-blocking way. For most modern browsers you can do this by adding the following attributes to your link tag:
<link href="mystyles.css" rel="preload" as="style">
Check async CSS loading on icanuse.com for the latest support stats.
If you need to support older browsers, use the loadCSS polyfill which does the same thing with javascript.
This method works by utilizing browser caching for the main stylesheet and maximizes rendering speed by inlining CSS that's required for the initial page load, very neat!
Use Minimal CSS for Additional Speed Gains
The most important thing you can do to stop CSS from slowing down your website is to use as little as possible. It's surprising how little CSS you need to make a beautiful-looking website.
I aim for a stylesheet small enough to be inlined on every page eliminating extra HTTP requests. This ensures my pages load with a single request (not including images).
Conclusion
So now you've learned all the methods of adding CSS to HTML and you've seen how they can work together to speed up your website.
I hope you've found this tutorial valuable.
Do you use <div>
tags to structure your website? Read this first: Replace Divs With Custom Elements For Superior Markup
Are you building a responsive website? The following articles may help you:
- Responsive Font Size (Optimal Text at Every Breakpoint)
- Responsive Padding, Margin & Gutters With CSS Calc
- Responsive Columns Layout System
- Responsive Banner Ads
Are IDs or classes better? See my guide for the answer: ID vs Class: Which CSS Selector Should You Use? (6 Examples).
![]()
“I've been developing websites professionally for over two decades and running this site since 1997! During this time I've found many amazing tools and services that I cannot live without.”
— Matthew James Taylor
I highly Recommend:
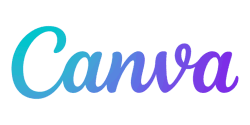
Canva — Best Graphic Design Software
Create professional graphics and social media imagery with an intuitive online interface.
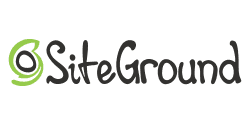
SiteGround — Best Website Hosting
Professional Wordpress hosting with 24/7 customer support that is the best in the industry, hands down!
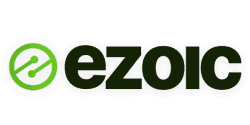
Ezoic — Best ad network for publishers
Earn more than double the revenue of Google Adsense. It's easy to set up and there's no minimum traffic requirements.
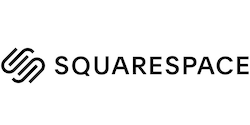
Squarespace — Best Website Hosting For Non-Developers
Easy-to-edit website templates, no coding needed. Full commerce and marketing features to run your business online.
See more of my recommended dev tools.